In this tutorial, we will explore how to implement quantity and total price calculations in a dynamic table using Vue.js. We’ll create a table where users can input the name of a fruit, its price, and the number of items they want to purchase. The total price for each fruit and the grand total for all items will be automatically calculated and displayed.
Implement Quantity and Total Price Calculations for Dynamic Tables in Vue js
To get started, make sure you have Vue.js installed or include it from a CDN in your HTML file. We’ll define our Vue.js app and its data structure to manage the table.
Here, we have a table with headers for Fruit Name, Item Price, Number of Items, and Total. We use Vue directives to bind the input fields to our data model.
Vue js: Calculate Quantity and Total Price in a Table
<div id="app">
<h3>Vue Js Calculate quantity and Total price in Table</h3>
<table>
<thead>
<tr>
<th class="fruit-name">Fruit Name</th>
<th class="item-price">Item Price</th>
<th class="num-items">Number of Items</th>
<th class="total">Total</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in items" :key="item.id">
<td>
<input type="text" v-model="item.fruitName" class="fruit-name-input" />
</td>
<td>
<input type="number" v-model="item.itemPrice" class="item-price-input" />
</td>
<td>
<input type="number" v-model="item.numItems" class="num-items-input" />
</td>
<td class="total-cell">{{ calculateTotal(item) }}</td>
</tr>
<tr>
<td colspan="3" class="grand-total-label">Grand Total</td>
<td class="grand-total">{{ grandTotal }}</td>
</tr>
</tbody>
</table>
</div>
Vue.js Data and Methods
In the script section of our HTML file, we define our Vue.js application and provide the data structure and methods necessary for our calculations
<script type="module">
const app = Vue.createApp({
data() {
return {
items: [
{ id: 4, fruitName: "Grapes", itemPrice: 8, numItems: 1 },
{ id: 5, fruitName: "Strawberries", itemPrice: 12, numItems: 5 },
{ id: 6, fruitName: "Mango", itemPrice: 7, numItems: 2 },
{ id: 7, fruitName: "Pineapple", itemPrice: 10, numItems: 3 },
{ id: 8, fruitName: "Watermelon", itemPrice: 20, numItems: 1 },
]
};
},
methods: {
handleItemChange(index, key, value) {
this.items[index][key] = value;
},
calculateTotal(item) {
return item.itemPrice * item.numItems;
},
},
computed: {
grandTotal() {
return this.items.reduce((total, item) => total + this.calculateTotal(item), 0);
},
}
});
app.mount("#app");
In the data section, we initialize an array of fruit items with their names, prices, and quantities. We also define methods for changing item values and calculating the total price for each fruit.
Calculating Total Price in Table
The calculateTotal
method multiplies the item’s price by the number of items, giving us the total cost for each fruit. The grandTotal
computed property uses reduce
to sum up the total prices of all fruits, providing the grand total for all items in the table
Output of Vue Js Calculate Quantity And Total Price In Table
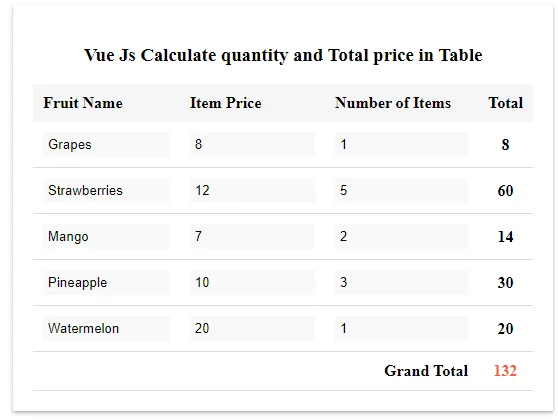
Conclusion
With this Vue.js implementation, users can easily input the name of a fruit, its price, and the number of items they want to purchase. The total price for each fruit and the grand total are dynamically calculated and displayed, providing a user-friendly experience for managing product orders in a table.